In this -third- part of our tutorial we are going to compile the GNU Debugger. It was ported to the Zilog Z80 architecture by Leonardo Etcheverry and is available from his git repository. The code is old and you need to apply some hacks for it to compile.
First install some additional tools. These are not part of the standard Ubuntu installation:
sudo apt-get install ncurses-dev flex bison
GNU Debugger also requires the texinfo package. But shall avoid compiling documentation because it is outdated and recent versions of texinfo are too strict for the job.Now fetch gdb-z80 source code by first cd-ing to your ~/dev folder and executing:
git clone https://github.com/legumbre/gdb-z80.git cd gdb-z80You are now ready to compile. Configure the package for cross debugging. Here is the command to do it:
./configure --enable-werror=no --target=z80-unknown-coffThe target of cross compilation is z80. The --enable-werror=no switch turns off error on warning behaviour on newer versions of gcc. The code is too old to pass without warnings and we don't want them to break the compilation.
Before running make we need means to avoid compiling documentation. The tool that we strive to avoid is makeinfo which is part of texinfo package. As you can remember we did not install it. So we are going to make the make think we have it by redirecting it to another tool which will do ... absolutely nothing ... and return success. It just so happens that unix has such a tool. It's called Wine. No, wait. It is called /usr/bin/true.
So here is how we call our make file
make MAKEINFO=trueWe apply the same trick to install gdb-z80 to destination folder
sudo make MAKEINFO=true installIf everything went according to our grand plan there are two new files in your /usr/local/bin folder.
ls /usr/local/bin z80-unknown-coff-gdb z80-unknown-coff-gdbtuiGrand! Now install your favourite GDB GUI. I recommend the Data Display debugger. It is absolutely archaic piece of technology and brings you back to the early days of unix when Motif roamed the earth. Just the right tool for ZX Spectrum development.
Install the DDD
sudo apt-get install dddAnd test our system by passing gdb-z80 to the Data Display Debugger using the --debugger option.
ddd --debugger z80-unknown-coff-gdb &
Yaay. You have a debugger for Z80 on your system. Next time we are going to dwelve into remote debugging and step through a program on your ZX Spectrum emulator.
Till then ... be patient.
Categories compilation , gdb , gnu , make , ubuntu , zx spectrum
So You Wanna Be a ZX Spectrum Developer? (Part II. The Template Application)
1 comment(s) at 5:23 AM
Let us compile'n'run stuff.
In part I. of this turorial you "gitted" yx repository to your local disk. If you followed the tutorial then your target folder was ~/dev/yx.
Open the terminal and set this as your current working folder. Go to tools subfolder (e.g. execute cd ~/dev/yx/tools). There are a bunch of useful tools inside but right now we are only interested in makezxbin. This is an upgraded makebin tool that enables us to use SDCC C compiler for ZX Spectrum development.
You are free to choose z88dk instead. But SDCC is -in my opinion- a more mature environment. It supports C99; has as advanced code optimizer; and produces gdb-z80 compatible symbolic information. z88dk on the other side has superior libraries and ZX Spectrum support.You can compile and deploy makezxbin like this:
cd makezxbin gcc makezxbin.c -o makezxbin sudo mv makezxbin /usr/bin/makezxbinCongratulations! You now have a complete suite of compiler tools needed to develop for ZX Spectrum.
Now go to the ~/dev/yx/apps/template folder and run make. This will create a template application which writes a value of 255 to the first byte of video memory. When it is run the result will be 8 pixel line in the first row of the screen:
The make file will also run the emulator and load your app.You can use this template application as a quick starter for most of your development projects. Let's analyse it.
First there is a pretty straightforward Makefile. Its main target is app.bin. The Makefile assumes that all *.c files ald all *.s files are part of our project. Thus if you add new source files - it will automatically consume them as part of our project.
For easier understanding here are the unconventional tricks of this Makefile:
- it uses your standard gcc (and not SDCC!) compiler to generate .depend file out of *.c and *.h source files for easier compilation,
- it puts crt0.s to 0x8000 and your program to 0x8032, so if you change crt0.s (increasing its length above 0x32 bytes) make sure you update your programs' start address,
- it uses our makezxbin tool to generate correct binary (makebin has a bug and can't be used for this purpose), and
- it uses SDCC all the way but at the end executes appmake +zx --binfile ./app.bin --org 32768 to generate ZX Spectrum tape. appmake is part of z88dk and this is one reason installing both development environments in part I. of this tutorial.
- stores current stack pointer and contents of all registers,
- jumps to start of GSINIT assembler section where the SDCC compiler places all global variables initialization code,
- establishes stack (1KB) and heap global symbols for our program,
- jumps to _main C function, and
- when the main concludes restores registers and stack and returns control to BASIC.
void main() { unsigned char * vmem = 16384; (*vmem)=255; }
You can examine the locations of your symbols (variables, functions) by opening crt0.map file after compilation.That's it for yoday. Enjoy your experimenting. Our next tutorial will be about debugging.
Categories c , compilation , gnu , make , ubuntu , zx spectrum
Here are some useful tips to help you start.
1. Download latest version of Ubuntu Desktop edition. I warmly recommend that you create a separate environment either by installing Ubuntu to a new partition or to a virtual machine. You see ... mid age crises ... come and go. While installed packages ... stay on your disks forever.
2. Download and install FUSE – the ZX Spectrum emulator. Since it lacks Sinclair ROM files, download and install these too.
sudo apt-get install fuse-emulator-gtk spectrum-roms
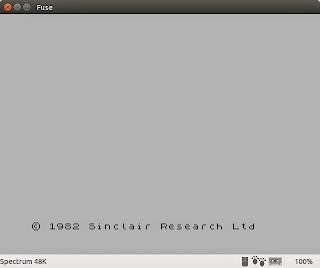
3. Download and install development suites.
sudo apt-get install sdcc z88dkYou don't actually need both C compilers. But each brings some handy tools that other can use. So install them both.
4. Download git and subversion so you'll be able to check out files from remote repositories
sudo apt-get install git subversion5. Install Z80 language definition for gedit.
5.1. Download z80.lang.zip and extract file z80.lang.
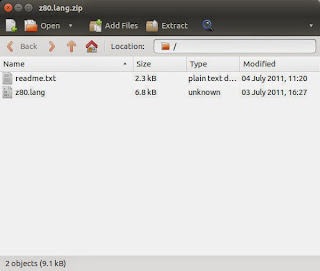
5.2. Modify it by changing this line:
<property name="globs">*.z80</property>to this line:
<property name="globs">*.s</property>You are telling gedit to treat all files with extension *.s as Z80 syntax files.
5.3. Now copy z80.lang into /usr/share/gtksourceview-3.0/language-specs folder.
6. Open terminal, create work folder and download the yx repository.
tomaz@jabba:~$ mkdir dev tomaz@jabba:~$ cd dev tomaz@jabba:~/dev$ git clone https://github.com/tstih/yx.git Cloning into 'yx'... remote: Reusing existing pack: 755, done. remote: Total 755 (delta 0), reused 0 (delta 0) Receiving objects: 100% (755/755), 5.60 MiB | 398.00 KiB/s, done. Resolving deltas: 100% (270/270), done. Checking connectivity... done. tomaz@jabba:~/dev$ cd yx tomaz@jabba:~/dev/yx$ ls apps buddy os README.md tools tomaz@jabba:~/dev/yx$_7. Extend gedit.
7.1. First open file ~/dev/yx/os/rom/crt0.s with gedit. If Z80 language is installed correctly you should see highlighted Z80 code.
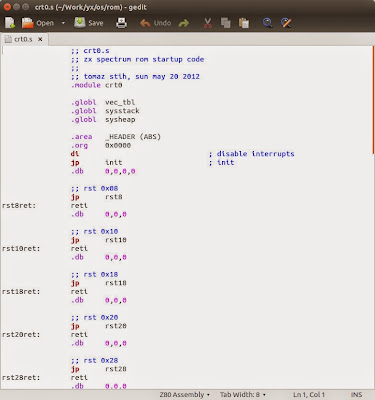
7.2. Now press F9 or select menu option View -> Side Panel. When the left pane appears change tab at the bottom of left pane from Documents to File Browser.
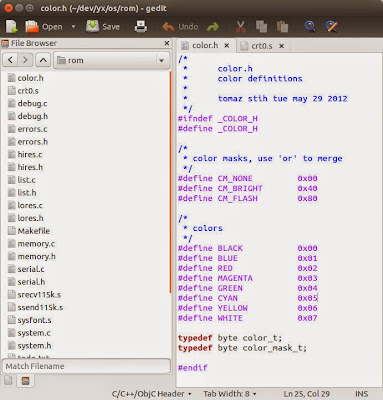
That's it for today. In second part of this tutorial we're going to compile and run stuff. Promise.
Categories c , compilation , gnu , ubuntu , zx spectrum